Have you ever wanted to spruce up the text output in your Bash scripts? Look no further! The echo -e
command allows you to add some flair to your output by incorporating special characters and formatting options.
Using echo -e
Using echo -e
is as simple as adding the -e
flag to your echo
command, followed by the string you want to output. For example:
echo -e "Hello, itvraag.nl!\\nWelcome to my Bash script."
This will output:
Hello, itvraag.nl!
Welcome to my Bash script.
Note the use of the \n
character to create a new line. This is just one example of the special characters that can be used with echo -e
.
Surprising Fact
Did you know that the echo
command is actually a shell builtin, rather than a separate program? This means that it is executed directly by the shell, rather than being called as an external program. This can make it faster and more efficient to use than other options for outputting text.
Coloring
To set the text color with echo -e
, you can use the \033[
escape sequence followed by a color code and the m
character. For example:
echo -e '\033[34mHello, itvraag.nl!\033[0m'
This will output the text “Hello, itvraag.nl!” in red. Here is a list of some common color codes:
31
: Red32
: Green33
: Yellow34
: Blue35
: Purple36
: Cyan37
: White
You can also set the text style using the \033[
escape sequence. For example:
echo -e '\033[1mHello, itvraag.nl!\033[0m'
This will output the text “Hello, itvraag.nl!” in bold. Here is a list of some common text style codes:
1
: Bold4
: Underline5
: Blink
You can combine color and style codes to create more complex formatting. For example:
echo -e '\033[36;1mHello, itvraag.nl!\033[0m'
This will output the text “Hello, itvraag.nl!” in bold cyan.
It’s important to remember to reset the text formatting after each output by using the \033[0m
code. This will ensure that your text formatting doesn’t carry over to subsequent outputs.
Here is an example script that demonstrates different text formatting options:
#!/bin/bash
echo -e '\033[31mHello, itvraag.nl!\033[0m'
echo -e '\033[32mHello, itvraag.nl!\033[0m'
echo -e '\033[33mHello, itvraag.nl!\033[0m'
echo -e '\033[34mHello, itvraag.nl!\033[0m'
echo -e '\033[35mHello, itvraag.nl!\033[0m'
echo -e '\033[36mHello, itvraag.nl!\033[0m'
echo -e '\033[37mHello, itvraag.nl!\033[0m'
echo -e '\033[1mHello, itvraag.nl!\033[0m'
echo -e '\033[4mHello, itvraag.nl!\033[0m'
echo -e '\033[5mHello, itvraag.nl!\033[0m'
echo -e '\033[36;1mHello, itvraag.nl!\033[0m'
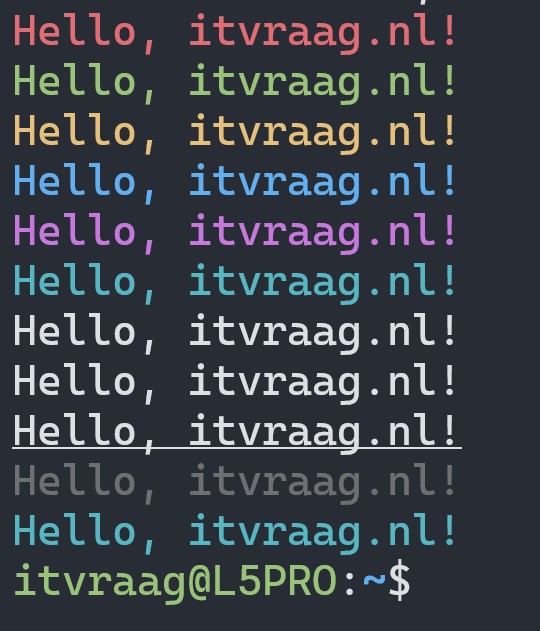
This will output the text “Hello, itvraag.nl!” in various colors and styles.
Key Takeaways
- The
echo -e
command allows you to add special characters and formatting options to your text output in Bash. - You can use
echo -e
in your Bash scripts to create more visually appealing or functional output. - The
echo
command is a shell builtin, which can make it faster to execute than external programs.
5 Tips for Increasing Productivity with echo -e
- Use special characters to add newlines, tabs, or other formatting options to your output.
- Use the
\033[
escape sequence to set the text color or style. For example,echo -e '\033[31mHello, itvraag.nl!\033[0m
‘ will output the text “Hello, itvraag.nl!” in red. - Use the
n
flag to suppress the newline that is added by default at the end of theecho
output. This can be useful for creating multi-line output without unnecessary blank lines. - Use the
e
flag in combination with the$'\n'
syntax to create a newline character. For example,echo -e "Line 1$'\n'Line 2"
will output “Line 1” and “Line 2” on separate lines. - Use the
>
operator to redirect the output ofecho
to a file, allowing you to save the output for later use or analysis.
Challenge
Now it’s your turn to try out echo -e
! Can you create a Bash script that outputs the following text, using echo -e
and at least one special character or formatting option?
Welcome to itvraag.nl!
Today is [insert current day of the week].
Hint: you can use the date
command to retrieve the current day of the week.